AI Created This Game
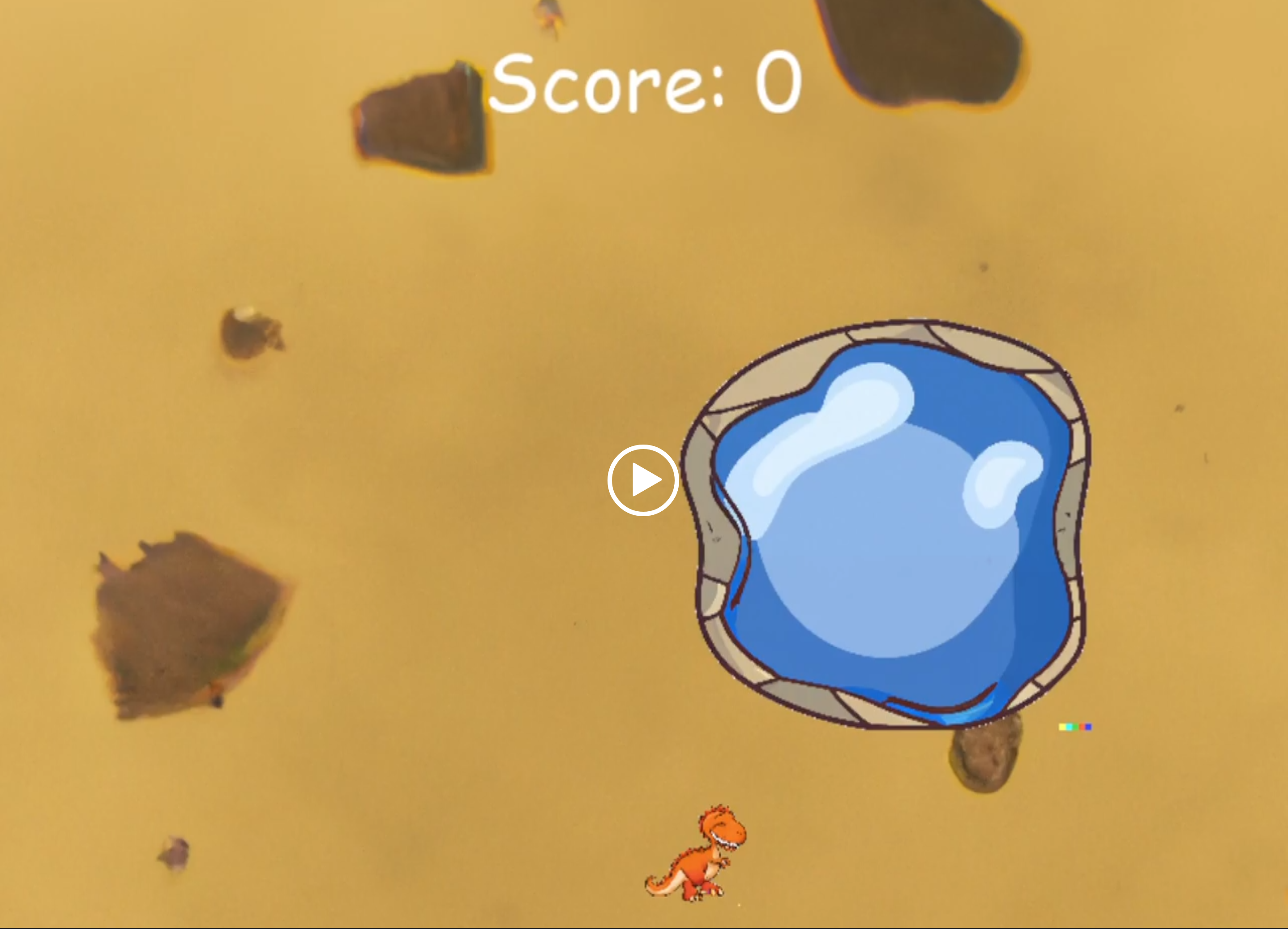
Machine learning is able to create computer code. It can create images. Put these together and have AI create an entire playable game. This video shows that AI-made game being played.
The AI generated this game from a single prompt after experimentation. No alterations and no fiddling with the code was done to make this game work. ChatGPT could not include images, so I generated the images it requested via the other OpenAI image generator, Dalle-2. I did have to edit the images to remove the white background, then I put them in the folder it wanted.
Machine learning generated
- The idea for this game
- The code for this game
- The art for this game
AI Generated Game Graphics
AI Generated Python Code
import pygame
import random
# Initialize Pygame
pygame.init()
# Set up the game window
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("Dinosaur Survival")
# Set up the background
background = pygame.image.load("prehistoric_background.png")
# Set up the font
font = pygame.font.SysFont("comicsansms", 50)
# Create the dinosaur sprite
dinosaur_image = pygame.image.load("dinosaur.png")
dinosaur_image = pygame.transform.scale(dinosaur_image, (64, 64))
dinosaur_x = 400
dinosaur_y = 500
dinosaur_speed = 0.1 # set the dinosaur speed to 0.1
# Generate food and water sources
food_image = pygame.image.load("food.png")
food_image = pygame.transform.scale(food_image, (96, 96))
water_image = pygame.image.load("water.png")
water_image = pygame.transform.scale(water_image, (256, 256))
food_x = random.randint(50, 750)
food_y = random.randint(50, 550)
water_x = random.randint(50, 750)
water_y = random.randint(50, 550)
# Set up the game loop
score = 0
running = True
while running:
# Check for user input
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# Update the dinosaur position
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
dinosaur_x -= dinosaur_speed
if keys[pygame.K_RIGHT]:
dinosaur_x += dinosaur_speed
if keys[pygame.K_UP]:
dinosaur_y -= dinosaur_speed
if keys[pygame.K_DOWN]:
dinosaur_y += dinosaur_speed
# Check for collision with food and water sources
if dinosaur_x < food_x + 96 and dinosaur_x + 64 > food_x and dinosaur_y < food_y + 96 and dinosaur_y + 64 > food_y:
# increment score and health, generate new food
score += 1
food_x = random.randint(50, 750)
food_y = random.randint(50, 550)
if dinosaur_x < water_x + 256 and dinosaur_x + 64 > water_x and dinosaur_y < water_y + 256 and dinosaur_y + 64 > water_y:
# increment score and health, generate new water
score += 1
water_x = random.randint(50, 750)
water_y = random.randint(50, 550)
# Draw the background and sprites
screen.blit(background, (0, 0))
screen.blit(dinosaur_image, (dinosaur_x, dinosaur_y))
screen.blit(food_image, (food_x, food_y))
screen.blit(water_image, (water_x, water_y))
# Draw the score
score_text = font.render("Score: " + str(score), True, (255, 255, 255))
score_rect = score_text.get_rect()
score_rect.center = (screen_width // 2, 50)
screen.blit(score_text, score_rect)
# Update the screen
pygame.display.update()
# Quit Pygame
pygame.quit()